- NULL Pointer
- Dangling Pointer
- Generic Pointers
- Wild Pointer
- Complex Pointers
- Near Pointer
- Far Pointer
- Huge Pointers
Saturday, 25 July 2015
TYPES OF POINTER
Pointer to array of pointer to string in c programming
Pointer to array of pointer to string in c programming
Pointer to array of pointer to string: A pointer to an array which contents are pointer to string.
Example of Pointer to array of pointer to string:
What will be output if you will execute following code?
#include<stdio.h>
Example of Pointer to array of pointer to string:
What will be output if you will execute following code?
#include<stdio.h>
int main(){
static char *s[3]={"math","phy","che"};
typedef char *( *ppp)[3];
static ppp p1=&s,p2=&s,p3=&s;
char * (*(*array[3]))[3]={&p1,&p2,&p3};
char * (*(*(*ptr)[3]))[3]=&array;
p2+=1;
p3+=2;
printf("%s",(***ptr[0])[2]);
return 0;
return 0;
}
Output: che
Explanation:
Here
ptr: is pointer to array of pointer to string.
P1, p2, p3: are pointers to array of string.
array[3]: is array which contain pointer to array of string.
Pictorial representation:

Note: In the above figure upper part of box represent content and lower part represent memory address. We have assumed arbitrary address.
As we know p[i]=*(p+i)
(***ptr[0])[2]=(*(***ptr+0))[2]=(***ptr)[2]
=(***(&array))[2] //ptr=&array
=(**array)[2] //From rule *&p=p
=(**(&p1))[2] //array=&p1
=(*p1)[2]
=(*&s)[2] //p1=&s
=s[2]=”che”
Multilevel pointers in c programming
Multilevel pointers in c programming
Multilevel pointers: A pointer is pointer to another pointer which can be pointer to others pointers and so on is know as multilevel pointers. We can have any level of pointers.
Examples of multilevel pointers in c:
Examples of multilevel pointers in c:
(1) What will be output if you will execute following code?
#include<stdio.h>
#include<stdio.h>
int main(){
int s=2,*r=&s,**q=&r,***p=&q;
printf("%d",p[0][0][0]);
return 0;
return 0;
}
Output: 2
Explanation:
As we know p[i] =*(p+i)
So,
P[0][0][0]=*(p[0][0]+0)=**p[0]=***p
Another rule is: *&i=i
So,
***p=*** (&q) =**q=** (&r) =*r=*(&s) =s=2
(2) What will be output if you will execute following code?
#include<stdio.h>
#include<stdio.h>
#define int int*
int main(){
int *p,q;
p=(int *)5;
q=10;
printf("%d",q+p);
return 0;
return 0;
}
Output: 25
Explanation: If you will see intermediate file you will find following code:
#include<stdio.h>
#include<stdio.h>
void main(){
int **p,q;
p=(int **)5;
q=10;
printf("%d",q+p);
return 0;
return 0;
}
Explanations:
Explanations:
Here q pointer and p is a number.
In c
Address + number = Address
So,
New address = old address + number * Size of data type to which pointer is pointing.
= 5 + 10 * sizeof (*int)
= 5+10*2 = 25.
Note. We are assuming default pointer is near. Actually it depends upon memory model.
POINTER TO A STRING, STRUCTURE ,
Pointer to array of string in c programming
Pointer to array of string: A pointer which pointing to an array which content is string, is known as pointer to array of strings.
What will be output if you will execute following code?
#include<stdio.h>
#include<stdio.h>
int main(){
char *array[4]={"c","c++","java","sql"};
char *(*ptr)[4]=&array;
printf("%s ",++(*ptr)[2]);
return 0;
return 0;
}
Output: ava
Explanation:
In this example
ptr: It is pointer to array of string of size 4.
array[4]: It is an array and its content are string.
Pictorial representation:

Note: In the above figure upper part of box represent content and lower part represent memory address. We have assumed arbitrary address.
++(*ptr)[2]
=++(*&array)[2] //ptr=&array
=++array[2]
=++”java”
=”ava” //Since ptr is character pointer so it
// will increment only one byte
Note: %s is used to print stream of characters up to null (\0) character.
Pointer to structure: A pointer which is pointing to a structure is know as pointer to structure.
Examples of pointers to structure:
Pointer to structure in c programming
Pointer to structure: A pointer which is pointing to a structure is know as pointer to structure.
Examples of pointers to structure:
What will be output if you will execute following code?
#include<stdio.h>
#include<stdio.h>
struct address{
char *name;
char street[10];
int pin;
}cus={"A.Kumar","H-2",456003},*p=&cus;
}cus={"A.Kumar","H-2",456003},*p=&cus;
int main(){
printf("%s %s",p->name,(*p).street);
return 0;
return 0;
}
Output: A.Kumar H-2
Explanation:
p is pointer to structure address.
-> and (*). Both are same thing. These operators are used to access data member of structure by using structure’s pointer.
DIFFERENCE BETWEEN int *ptr[100] and int (*int)[100] and pointer to 2d and 3d array
int (*ptr) arr[1000] - is a pointer to an array of 1000 elements or 10000 is the size of the column and rows can be dynamically created
int *arr[10000] is an array of pointer contains 10000 pointers or 10000 is the size of the row and column will be dynamically created
declaring pointer to a 2d array
int arr[100][100]
int (*ptr)[100]=arr;
ac-- value 2d array
arr[i][j]=(*(arr+i))[j] or *((*arr+i)+j)
ex-
arr[0][0]=(*(arr))[0] or *((*arr)+0)
Friday, 24 July 2015
DIFFERENCE BETWEEN POINTER AND ARRAY POINTER
Difference between array and pointer:
- Only for pointers, you need to explicitly allocate and deallocate memory, unless pointing to variable. This will be in heap unlike stack as in case for arrays.
- For pointer, memory to which it points can be resized using realloc. Array cannot be resized.
- You can take address of the pointer variable. Address of array will give you address for first element of that array. This is because array is just a name to a set of memory locations, whereas pointer is a variable.
- Sizeof pointer is always fixed. 8 byte for 64 bit machines and 4 bytes for 32 bit machine to store the start address( 64 bit or 32 bit ) of memory. Sizeof array is strlen of array + 1.
- array pointer cant be incremented but normal pointer can be increment . ex- int *pt; pt++ valid . but int ar[100] arr++ this in invalid ;
- c does not allow array names tobe use as an lvalue . hence array name cant be apear on the left hand side of the assigment operator . ie int arr[100]; int *ptr; arr=ptr invalid .
- array cant be assign to another array , but point can be assign to another pointer of same type ex- int arr[100],brr[100] arr=brr invalid
- main difference is the return value of the address operator address operator return the address of the operator but when address operator is applied to the array name it will give the same value as array referance without the operator i.e int arr[1000] than arr is same as &arr.
- last difference is that when we applied sizeof() operator to the array it will give the size of all bytes allocated for the array but for normal pointer it willgive the byte of pointer variable allocation (2 , 4 , 8 dependent on matchine)
SIZE OF DATA TYPE
- Below table gives the detail about the storage size of each C basic data type in 16 bit processor.
Please keep in mind that storage size and range for int and float datatype will vary depend on the CPU processor (8,16, 32 and 64 bit)
S.No | C Data types | storage Size | Range |
1 | char | 1 | –127 to 127 |
2 | int | 2 | –32,767 to 32,767 |
3 | float | 4 | 1E–37 to 1E+37 with six digits of precision |
4 | double | 8 | 1E–37 to 1E+37 with ten digits of precision |
5 | long double | 10 | 1E–37 to 1E+37 with ten digits of precision |
6 | long int | 4 | –2,147,483,647 to 2,147,483,647 |
7 | short int | 2 | –32,767 to 32,767 |
8 | unsigned short int | 2 | 0 to 65,535 |
9 | signed short int | 2 | –32,767 to 32,767 |
10 | long long int | 8 | –(2power(63) –1) to 2(power)63 –1 |
11 | signed long int | 4 | –2,147,483,647 to 2,147,483,647 |
12 | unsigned long int | 4 | 0 to 4,294,967,295 |
13 | unsigned long long int | 8 | 2(power)64 –1 |
Q WHY ONLY SIZE OF INT DATA TYPE VARIES WITH DIFFERENT ARCHITECTURE
The smaller types have the advantage of taking up less memory, the larger types incur a performance penalty. Variables of type int store the largest possible integer which does not incur this performance penalty. For this reason, int variables can be different depending what type of computer you are using.
OR
Size of data types in c programming language turbo C and GCC compilers
Size of data types in the 16 bit compilers, like TURBO c++ 3.0, Borland c++ etc:
Size of data types in the 32 bit compilers. Example: LINUX gcc compiler, Turbo c 4.5 etc:
union basic
C Programming Unions
Unions are quite similar to the structures in C. Union is also a derived type as structure. Union can be defined in same manner as structures just the keyword used in defining union in union where keyword used in defining structure was struct.
union car{ char name[50]; int price; };
Union variables can be created in similar manner as structure variable.
union car{ char name[50]; int price; }c1, c2, *c3; OR; union car{ char name[50]; int price; }; -------Inside Function----------- union car c1, c2, *c3;
In both cases, union variables c1, c2 and union pointer variable c3 of type
union car
is created.Accessing members of an union
The member of unions can be accessed in similar manner as that structure. Suppose, we you want to access price for union variable c1 in above example, it can be accessed as
c1.price
. If you want to access price for union pointer variable c3, it can be accessed as (*c3).price
or as c3->price
.Difference between union and structure
Though unions are similar to structure in so many ways, the difference between them is crucial to understand. This can be demonstrated by this example:
#include <stdio.h>
union job { //defining a union
char name[32];
float salary;
int worker_no;
}u;
struct job1 {
char name[32];
float salary;
int worker_no;
}s;
int main(){
printf("size of union = %d",sizeof(u));
printf("\nsize of structure = %d", sizeof(s));
return 0;
}
Output
size of union = 32 size of structure = 40
There is difference in memory allocation between union and structure as suggested in above example. The amount of memory required to store a structure variables is the sum of memory size of all members.
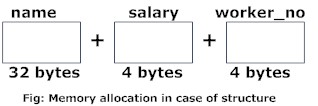
But, the memory required to store a union variable is the memory required for largest element of an union.
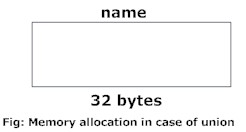
What difference does it make between structure and union?
As you know, all members of structure can be accessed at any time. But, only one member of union can be accessed at a time in case of union and other members will contain garbage value.
#include <stdio.h> union job { char name[32]; float salary; int worker_no; }u; int main(){ printf("Enter name:\n"); scanf("%s",&u.name); printf("Enter salary: \n"); scanf("%f",&u.salary); printf("Displaying\nName :%s\n",u.name); printf("Salary: %.1f",u.salary); return 0; }
Output
Enter name Hillary Enter salary 1234.23 Displaying Name: f%Bary Salary: 1234.2
Note: You may get different garbage value of name.
Why this output?
Initially, Hillary will be stored in
u.name
and other members of union will contain garbage value. But when user enters value of salary, 1234.23 will be stored in u.salary
and other members will contain garbage value. Thus in output, salary is printed accurately but, name displays some random string.Passing Union To a Function
Union can be passed in similar manner as structures in C programming. Visit this page to learn more about: How structure can be passed to function in C programming?
Difference between structure and union in C:
S.no | C Structure | C Union |
1 | Structure allocates storage space for all its members separately. | Union allocates one common storage space for all its members. Union finds that which of its member needs high storage space over other members and allocates that much space |
2 | Structure occupies higher memory space. | Union occupies lower memory space over structure. |
3 | We can access all members of structure at a time. | We can access only one member of union at a time. |
4 | Structure example: struct student { int mark; char name[6]; double average; }; | Union example: union student { int mark; char name[6]; double average; }; |
5 | For above structure, memory allocation will be like below. int mark – 2B char name[6] – 6B double average – 8B Total memory allocation = 2+6+8 = 16 Bytes | For above union, only 8 bytes of memory will be allocated since double data type will occupy maximum space of memory over other data types. Total memory allocation = 8 Bytes |
Subscribe to:
Posts (Atom)